This guide will show you how to create a lead generation tool from provided sample code, using AWS serverless technologies and Plunk Home Value API.
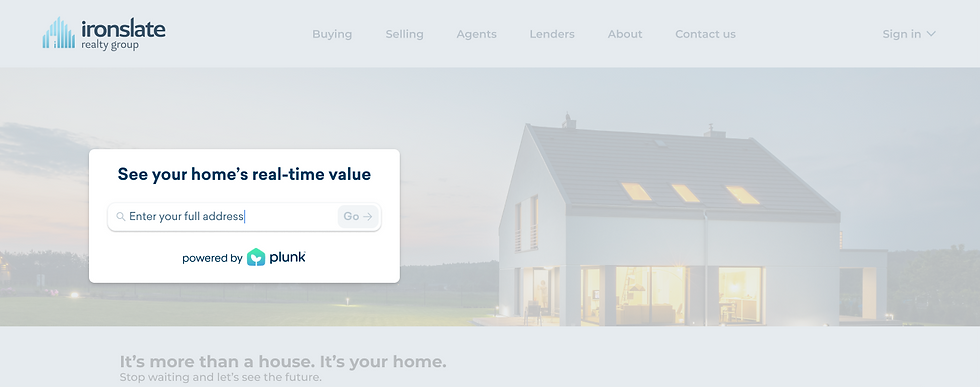
Overview
The lead generation tool you’ll develop allows a prospective client to check their home’s value, providing the agent with the lead’s contact info for follow-up later. Sample code is provided to help you get started with a React frontend and AWS Amplify backend. When finished, you’ll have a tool hosted in AWS that will rebuild and redeploy automatically when you push changes to the connected GitHub repository.
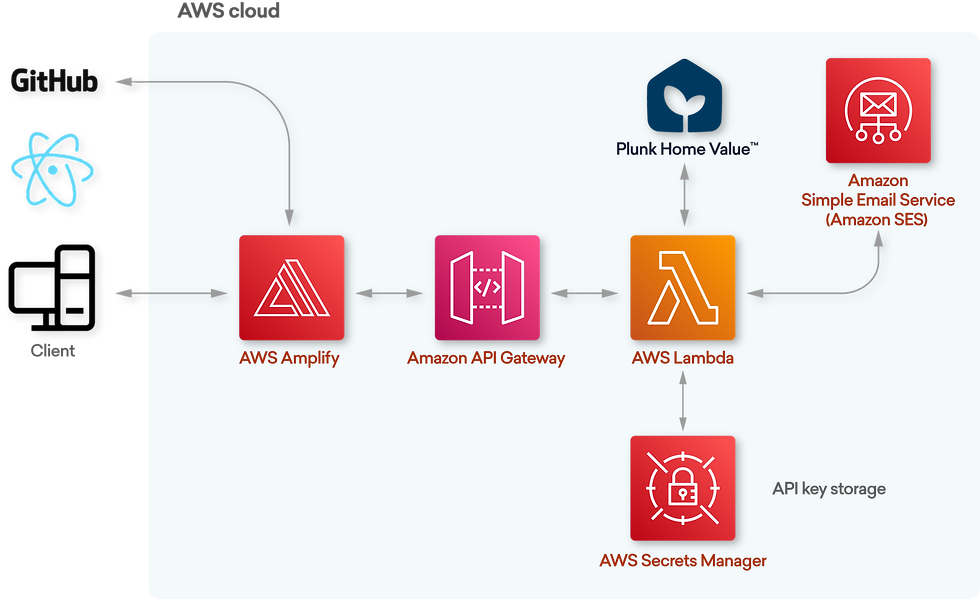
Why create a lead generation tool?
With growing competition in the residential real estate market and limited supply, generating quality leads is vital for an agent’s success.
Generating leads means finding potential clients who may be interested in buying or selling properties, which is the primary source of income for real estate agents. Therefore, effective lead generation is crucial for agents to grow and maintain their businesses.
Equipped with tools that generate high-quality leads, REALTORS® can cultivate financial success and, more importantly, deliver genuine value to buyers and sellers who are navigating critical decisions.
Why use AWS serverless technologies?
There are a few benefits that can make your life as a developer much easier when using AWS.
Amplify removes the tedious DevOps and configuration burden when trying to deploy an app. The deployment process with Amplify is alarmingly easy and quick.
The project is easier to scale, as you don’t need to focus on provisioning hardware. AWS takes care of that for you.
AWS Lambda scales automatically, which allows performance to remain consistently high as the event frequency increases. Also, since your code is stateless, Lambda can start as many instances as needed without lengthy deployment and configuration delays.
AWS services are well documented and popular in the developer community should you need help with anything.
Requirements
There are a few benefits that can make your life as a developer much easier when using AWS.
Time to complete: ~2 hrs
Sample code and API key (request from Plunk)
AWS account
Technologies used:
React
GitHub
Node.Js
npm
Plunk Home Value API
AWS Amplify
Amazon API Gateway
AWS Lambda
AWS Secrets Manager
Amazon Simple Email Service
Let's get started
First, you will need to create a new repository in GitHub. You will want to make sure not to add a README.md file or make the first commit yet. Once you have created the new repository, leave the tab open on the page that prompts you to make your first commit. We will come back to this later in order to copy the HTTPS URL.
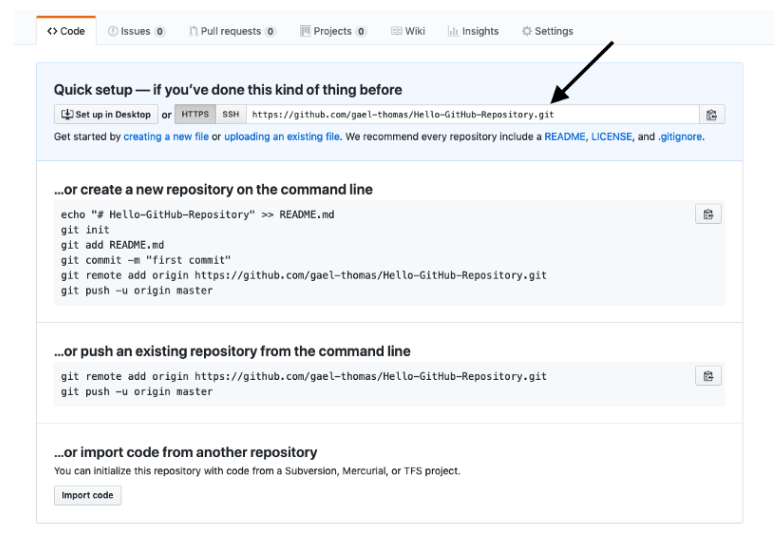
Setting up the sample code on your local machine
Next, you will need the zip file—provided by Plunk—for the sample code. Unzip the file and open the project in an IDE of your choosing. I chose to use VS Code. For the next step you will need to make sure you have Node.Js installed on your local machine.
Next, while in the root of the project, run npm install in your CLI to install all dependencies.
npm install
Now, change directories (cd) into amplify/backend/function/plunkLeadGenLambda/src and again run npm install.
npm install
Push your code into your new GitHub repository
You are now ready to push your project into the new GitHub repository you recently created. Later, AWS will be able to sync with this code repository in GitHub before deployment.
Copy the HTTPS URL for your repository to use in the third command listed below. The URL can be found on the GitHub tab we left open after creating the repository.
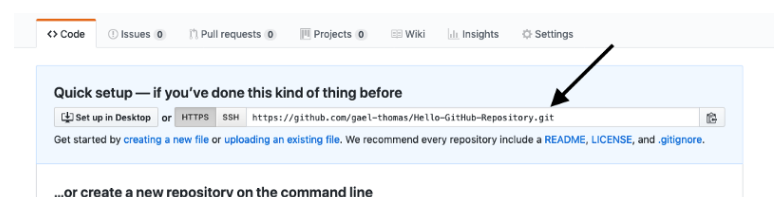
Then, use these commands in your CLI to make your first commit and push your code into the repository:
git init -b main
git add .
git commit -m “first commit”
git remote add origin <remote_https_url_for_github_repo>
git remote -v (verifies the new remote URL)
git push -u origin main
The code from your project should now be saved in your GitHub repository. If you make any changes in the future to the frontend code make sure you push those changes to your GitHub repository.
Configure the AWS Amplify backend
Install AWS Amplify CLI if you don’t have it installed already.
npm install -g @aws-amplify/cli
Next, run amplify configure in your CLI to configure the AWS Amplify backend.
amplify configure
Next, sign in to your AWS account and specify the region.
Next, go to Identity and Access Management (IAM) in your AWS Console to complete the IAM user creation process:
Go to Users.
Click Add users.
Create a User name and click Next.
You will want to click Attach policies directly.
AdministratorAccess-Amplify is the only policy you need to select for this user. Click Next.
Click Create user.
In the Users dashboard, click on the user you just created.
Click on the Security credentials tab**.**
In the Access keys section, click Create access key.
Choose Command Line Interface and click Next.
Create a Description tag value like “myAccessKey” and DO NOT click done. Save the Access key and Secret access key somewhere you can view them again outside of AWS.
Go back to your terminal and hit enter on your keyboard, if you haven’t already.
Type in your Access key when prompted and hit enter.
Type in your Secret Access key when prompted and hit enter.
Type in an AWS profile name you’d like to use and hit enter.
Next, cd into the root of the project and run amplify init to begin completing the initialization process:
amplify init
Name a new environment. I suggest just using the default “dev” for experimentation.
Choose your default editor. I chose VS Code.
Select the authentication method you want to use (this tutorial used AWS Profile).
Choose the AWS profile you wish to use
Now you are ready to commit and push the changes you have made to the project in AWS. To commit the changes, run amplify push. This may take a couple of minutes the first time. Don’t forget to do this in the future if you make changes to the Amplify backend.
amplify push
Lastly, you will be prompted to add a value for the SES_EMAIL env variable. Please choose an email address from which you will be sending home valuations to leads. This can be a test email for now, however, you will need to be able to access the email in next step.
Configure Amazon Simple Email Service (SES)
SES is used to automatically send home valuation information to leads from a verified email. You will need to verify your email on the SES page within you AWS console.
Go to your AWS console by running amplify console in your terminal:
amplify console
Go to the SES page by searching Amazon Simple Email Service in your AWS console.
Complete the identity creation process:
Go to Create identity.
Choose Email address as the identity type.
Type the email address from which you will be sending emails to leads.
Click Create identity.
You will be sent an email to verify your email address.
Once verified, copy the Amazon Resource Name (ARN) associated with the email. You will use it in the next section.
Please note, when first creating a new identity(email) in SES, this identity will be in sandbox mode. This means you won’t actually be able to send emails to users until you are granted production access.
To request production access, visit the account dashboard in your Amazon SES.

Once production access is granted, go to
yourProject/amplify/backend/function/plunkLeadGenLambda/src/app.js
and on line 125 and 126 update the values of the ToAddresses to be the user-inputted email address (emailRecipient). This will ensure you are sending emails to the lead’s input email.
Update code with correct email ARN value
After creating the email identity, you will need to update your code with the correct ARN value.
In your code, go to the plunkLeadGenLambda-cloudformation-template.json file to update the ARN value. On line 208 as the first value in the Fn::Sub array, replace yourArnValue with your ARN value from the previous step.
Save and push this change to your project in AWS by running amplify push .
amplify push
Store your API key with AWS Secrets Manager
You’ll want to store your API key using AWS Secrets Manager to prevent checking it into GitHub. There, it could be seen by others, if it isn’t a private repository. We don’t want to give anyone access to the key EXCEPT you.
Go to the AWS console:
amplify console
Search for AWS Secrets Manager in your AWS console and complete the process to store a new secret:
Click “store new secret” button.
Choose “Other” type of secret.
Use API_KEY_VALUE for the key name and your API key for the value of the key name.
Use phv-api-keyas your secret name.
Click Next until you are able to create the key.
View your secret and make sure to copy its ARN value for the next step.
After creating the secret you will need to update your code with the correct ARN value.
Go to the plunkLeadGenLambda-cloudformation-template.json file to update the ARN value. On line 167 as the first value in the Fn::Sub array, replace yourArnValue with your secret ARN value from the previous step.
Save and push this change to your project in AWS by running amplify push.
amplify push
Test the app locally
Before testing the tool locally, double-check to make sure you have committed all changes in your code to the GitHub repository you created for this project.
To test the tool locally, you will use an npm script in the CLI to start the dev server and get it running in a fresh tab in your browser.
npm start
Once it is up and running in the browser, go through the flow and make sure it is working as expected.
Deploy the app
Now that the tool is successfully running in your local environment, it is time to deploy it using AWS.
Go to the AWS console:
amplify console
Go to AWS Amplify and complete the process to deploy the app:
Go to Hosting environments.
Choose to connect with your GitHub repository and authorize AWS to integrate with your GitHub. Authorize Amplify to connect with all repositories or the specific repository and select your preferred option to link your AWS account.
Finally, deploy the app.
Please note, anytime you make changes to your GitHub repository that is connected to AWS, a new build and redeployment will occur automatically.
Here is another video tutorial on creating and deploying an app with AWS amplify that helped me during this process.
Projected costs for 1M leads / year
You may be wondering what creating an app like this will cost. Below is a breakdown of the expenses associated with using the AWS, assuming you will generate one million leads per year (~83k per month). If you are interested in pricing for accessing the Plunk Home Value API, please reach out to us here (link).
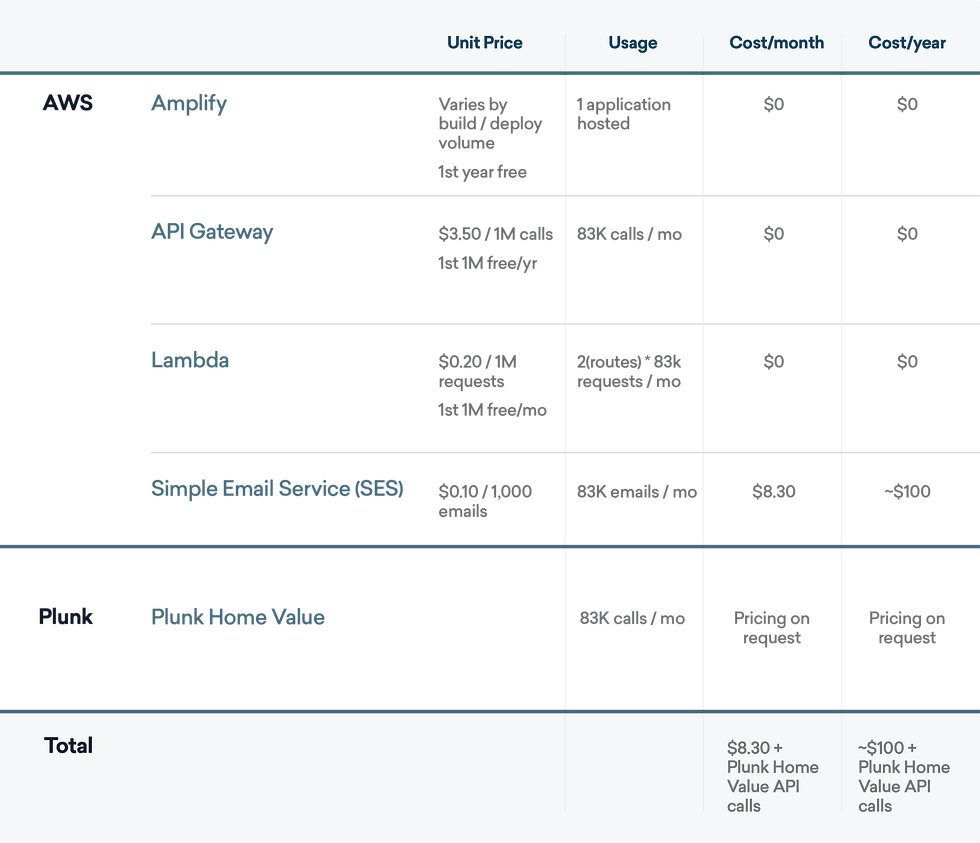
Additional resources
Once you get your lead generation tool up and running, you may want to make further changes to adapt it to fit your own needs and design style. Below are some videos that helped me with configuring different aspects of the project and may aid in your efforts.
Thank you
I appreciate you taking the time to follow along. If you have any questions on this example or about Plunk, please feel free to reach out to me at steve@getplunk.com.